The dotLottie web player, powered by the ThorVG rendering engine, offers consistent animation quality across all platforms. ThorVG's cross-platform capabilities ensure that animations look the same regardless of where they are viewed.
In this blog post, we'll focus on the dotlottie-web player, an isomorphic JavaScript library that renders and plays dotLottie and Lottie animations. We'll specifically demonstrate how to use dotlottie-web in a Node.js environment to easily convert dotLottie animations into Graphics Interchangeable Format (GIF).
Setting Up the Environment
Prerequisites
- Ensure you are using Node.js version 18 or higher.
Installation
First, install dotlottie-web
:
npm install @lottiefiles/[email protected]
For canvas implementation in Node.js, @napi-rs/canvas
is recommended for its performance:
npm install @napi-rs/canvas
And don't forget the GIF encoder:
npm install gif-encoder
Creating the dotLottie2gif.ts script
Create a new file named dotLottie2gif.ts
. This file script will be our main tool for converting animations to GIFs.
Step 1: Import and Initialize
In dotLottie2gif.ts
, start by importing the necessary modules and setting up your canvas and GIF encoder:
import { createCanvas } from "@napi-rs/canvas";
import GIFEncoder from "gif-encoder";
import { DotLottie } from "@lottiefiles/dotlottie-web";
import fs from "fs";
const WIDTH = 200;
const HEIGHT = 200;
// Create a canvas with the specified width and height
const canvas = createCanvas(WIDTH, HEIGHT);
// Create a GIF encoder with the same dimensions as the canvas
const gif = new GIFEncoder(WIDTH, HEIGHT);
Step 2: Configure dotLottie web
Set up the DotLottie
instance with the necessary configurations:
// Initialize dotLottie with specific settings
const dotLottie = new DotLottie({
loop: false, // Prevent looping for single playback
useFrameInterpolation: false, // Disable frame interpolation as it's not supported by gif
canvas: canvas as unknown as HTMLCanvasElement, // Canvas for rendering
// Credit goes to -> <https://lottiefiles.com/animations/celebration-lolo-w318l83FuX>
src: "<https://lottie.host/9ac9c440-c19e-4ac9-b60e-869b6d0ef8cb/7h97gYMCNE.lottie>", // URL to lottie or dotLottie animation
autoplay: true, // Start playback upon loading
});
Step 3: Handle Events
In the dotLottie2gif.ts
script, we need to handle three events to manage the GIF conversion process:
- Load Event: Triggered when the dotLottie player has loaded the animation. Here, we initialize the GIF encoding process. We create a writable stream for the output GIF file and configure the GIF encoder with appropriate settings, like frame delay, based on the animation's frame rate.
- Frame Event: In this event, we capture the current frame from the canvas context and add it to the GIF encoder. This is where the conversion from an animation frame to a GIF frame happens.
- Complete Event: When this event is triggered, we finalize the GIF file by calling
gif.finish()
, which completes the encoding process and closes the file stream.
dotLottie.addEventListener("load", () => {
const outputGifFile = fs.createWriteStream("./animation.gif"); // Stream for output GIF file
gif.pipe(outputGifFile); // Pipe the encoder output to the file stream
gif.writeHeader(); // Write the initial header for the GIF
// Calculate frame delay from animation frame rate
const fps = dotLottie.totalFrames / dotLottie.duration;
gif.setDelay(1000 / fps); // Set frame delay in milliseconds
gif.setRepeat(0); // Configure repeat setting for the GIF (0 for repeat)
});
dotLottie.addEventListener("frame", () => {
const ctx = canvas.getContext("2d"); // Get the 2D context of the canvas
const frameBuffer = ctx.getImageData(0, 0, WIDTH, HEIGHT).data; // Extract frame data
gif.addFrame(frameBuffer); // Add the current frame to the GIF
});
dotLottie.addEventListener("complete", () => {
gif.finish(); // Finish the GIF encoding and close the file stream
});
Step 4: Running the Script
Execute dotLottie2gif.ts
with:
npx tsx dotLottie2gif.ts
This generates animation.gif
file.
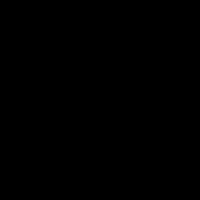
If you're interested in hands-on learning, feel free to play around and test the dotlottie2gif script we've created earlier. You can access it by exploring the provided devbox here.
The dotLottie player allows for other customization like the background color, speed and segments. Check the README in the dotlottie-web
repository for detailed information on API usage and configurations.
Conclusion
The dotLottie web player is an isomorphic JavaScript library that can run in both web browsers and Node.js, thanks to the ThorVG cross-platform rendering engine.
It can be easily integrated into various JavaScript frameworks. If you're curious, have a look at our React wrapper and our web component wrapper.
Don't forget to check out dotlottie-web on GitHub today. Feel free to contribute to its development and remember to give us a star if you find it useful!