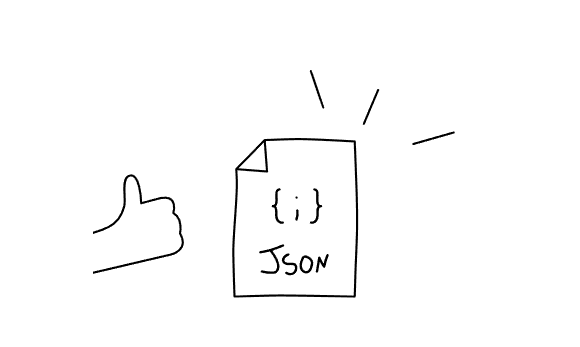
This article assumes that you are familiar with Lottie and targets the developer audience. Over the past few years, Lottie has gained traction in popularity, and the demand for tools to manipulate Lottie, as well as tools to work with Lottie, has increased.
Tooling in the Lottie-verse
The first step to building tools around the Lottie JSON Object is to be able to understand the structure of the Lottie JSON. The Lottie JSON object is based on the structure of animation and composition within Adobe After Effects CC coupled with modifications as needed by Hernan Torrisi the creator of the bodymovin plugin that facilitated the export of animations from After Effects to JSON.
Problems in developing Lottie tools
There are a few problems that come to mind given my experience in building tools around Lottie such as the current Lottie editor at LottieFiles as well as other internal tools.
First up is the fact that key-value pairs use abbreviations in the JSON object and therefore make understanding via reading the JSON object nearly impossible except for maybe a few properties in the outer layer of the object. Understandable since we want to keep the file size as small as possible however this makes it really difficult to map which features of After Effects map onto which property in the JSON object considering we have limited documentation. Trying to make sense of the object below is definitely a herculean feat.
{"v":"5.6.2","fr":30,"ip":0,"op":105,"w":1000,"h":500,"nm":"Olove","ddd":0,"assets":[],"layers":[{"ddd":0,"ind":1,"ty":4,"nm":"Layer 3 Outlines","sr":1,"ks":{"o":{"a":1,"k":[{"i":{"x":[0.667],"y":[1]},"o":{"x":[0.333],"y":[0]},"t":62,"s":[0]},{"t":68,"s":[100]}],"ix":11},"r":{"a":1,"k":[{"i":{"x":[0.667],"y":[1]},"o":{"x":[0.167],"y":[0.167]},"t":45,"s":[90]},{"t":75,"s":[0]}],"ix":10},"p":{"a":1,"k":[{"i":{"x":0.667,"y":1},"o":{"x":0.167,"y":0.167},"t":45,"s":[1258.012,275.48,0],"to":[-76,0,0],"ti":[76,0,0]},{"t":75,"s":[802.012,275.48,0]}],"ix":2},"a":{"a":0,"k":[66.421,71.882,0],"ix":1},"s":{"a":0,"k":[100,100,100],"ix":6}},"ao":0,"shapes":[{"ty":"gr","it":[{"ind":0,"ty":"sh","ix":1,"ks":{"a":0,"k":{"i":[[0,0],[20.799,0],[2.86,-21.319]],"o":[[-2.082,-20.28],[-19.242,0],[0,0]],"v":[[35.491,-9.753],[0.651,-45.891],[-34.97,-9.753]],"c":true},"ix":2},"nm":"Path 1","mn":"ADBE Vector Shape - Group","hd":false}],"nm":"Group 1","np":4,"cix":2,"bm":0,"ix":1,"mn":"ADBE Vector Group","hd":false}],"ip":0,"op":240,"st":0,"bm":0}],"markers":[]}
The second hurdle can be easily overcome. Most often developers don't have After Effects knowledge, we don't really know what a “pre-composition” is nor other terminologies used in the motion design world. Easily solvable by going through After Effects documentation and searching through other articles and tutorials on the web.
The solution
However, to take tooling in the Lottie-verse to the next level, we need to document exactly which key and value inside the JSON object refer to which After Effects property/effect or feature, followed by documenting and explaining exactly what the property/effect or feature does in After Effects in layman's terms. This will alleviate the burden of developers having to change key-value pairs in the JSON object to understand how it changes the animation and also alleviates the burden of having to research After Effects features from different parts of the internet. The Lottie-JS library is an attempt to solve both of those problems as mentioned above. Refer to full documentation below.
https://docs.lottiefiles.com/lottie-js/
Usage
Install
yarn add @lottiefiles/lottie-js
Get started
import { Animation } from '@lottiefiles/lottie-js';
async function loadAnimation() {
// Create Lottie instance
// (you can also use Animation.fromJSON method if you already have the Lottie JSON loaded)
const anim = Animation.fromURL('https://assets1.lottiefiles.com/packages/lf20_u4j3xm6r.json');
// Print some data of the animation
console.log('Frame Rate', anim.frameRate);
console.log('Number of Layers', anim.layers.length);
console.log(anim.getColors());
// Manipulate animation
anim.name = 'Woohoo';
anim.width = 512;
anim.height = 512;
// Get the new JSON
const woohooLottie = JSON.stringify(anim);
console.log(woohooLottie);
}
Promise.resolve(loadAnimation);
The goal
The library consists of methods to map the Lottie JSON to a more structured object model and to allow interactions with layers/shapes/properties as well as manipulate them. The goal is to fully map the Lottie object model and add enough helper methods to the library such that the complexity of the Lottie JSON is abstracted away thereby allowing the whole of Lottie-verse to grow.
Where we are at
As the library stands now, the structure is as follows in a nutshell. anAnimation class acts as the base class that contains all of the given information about Lottie. Inside of which contain Layer & Asset base classes, each of which can have different types. Each Layer in addition to its simple properties such as height, width, etc can have more complex properties namely Anchor, Opacity, Rotation, Position, and Scale (AORPS) all of which are classed under Property.
Limitations
- All property types currently exist under the one Property base class, however, many different property types exist in addition to the ones mentioned above (AORPS) and the objects come in various forms depending on the types.
- Moreover, all property types can be animated and keyframe. An elegant way is needed to manage the timelines of property types on all property types across all the different layers. This has not been implemented and the key-frame class is a completely separate class. This class however handles the bezier curves. A Bézier curve is a parametric curve frequently used in computer graphics and related fields. Bézier curves are also used in animation as a tool to control motion. In animation applications, such as Adobe Flash and Synfig, Bézier curves are used to outline, for example, movement.
Where do we go from here?
The team at LottieFiles is small and manpower is limited as is time, we are open-sourcing this project in hopes that we as a community can grow the tooling eco-system surrounding Lottie. This project is a good first step to properly document as well as create API methods that will allow developers to build cool new tech that can interact with Lottie animations. Once the object model is sufficient and matured after overcoming the limitations as mentioned above we can then proceed to abstract a step further and write in helper methods to manipulate as well as view properties in a manner that is usable for tooling. Current documentation is seen here [click here] and current API methods to interact with Lottie are seen here [click here] and will serve as a good reference. A few bullets on where we can start:
- Changing property class to a base property class with subtypes.
- Adding property types and adding in all the types and writing the classes.
- Figuring out a mechanism to handle keyframing of property types.
- Implementation of this mechanism/abstraction.
How to develop & contribute
You’ll need Node.js version 12 or 14 to build the library. If you’re using nodenv
, read the nodenv
docs for instructions on switching Node.js versions.
Once you’ve installed Node.js or Yarn, open Terminal and run the following:
git clone [email protected]:LottieFiles/lottie-js.git
cd lottie-js
yarn install
yarn test
yarn build
Do read our contribution guidelines [click here] before creating pull requests and we welcome you to work with us in the expansion of the Lottie-verse.